Published
- 3 min read
How to Remove Undefined from an Array in JavaScript?
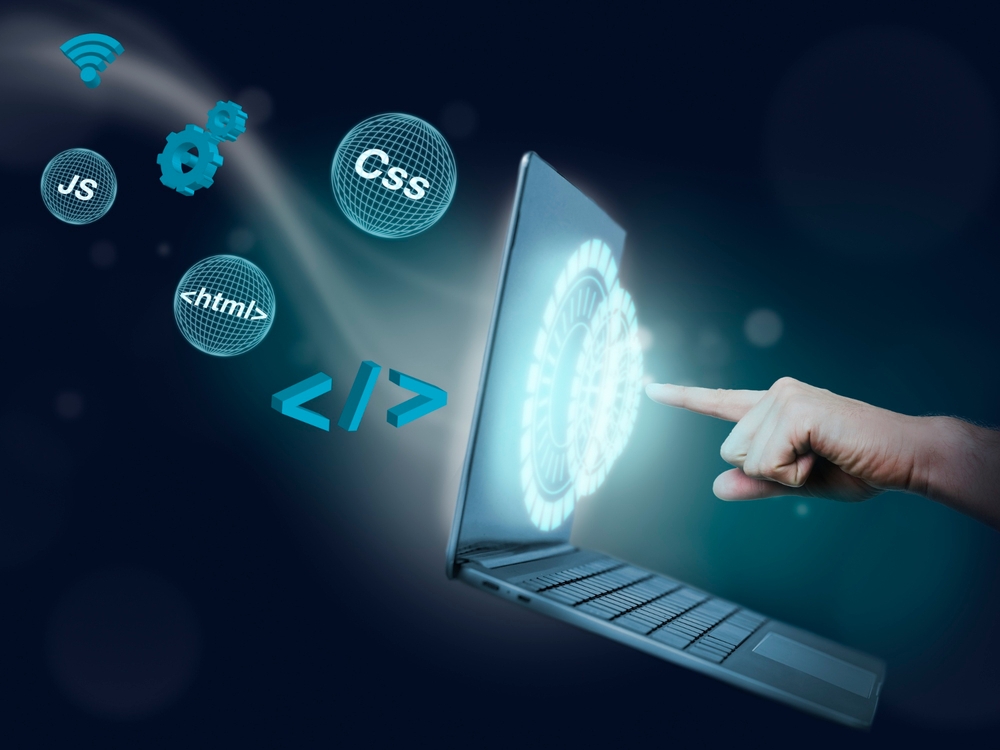
Hello! Are you learning JavaScript? Don’t worry! I’m here to explain things in simple, easy words. Today, we’ll learn how to remove undefined
from an array in JavaScript step by step. 😊
What is Undefined?
In JavaScript, undefined
means that something exists but doesn’t have a value yet. Let me give you an example:
let myVar
console.log(myVar) // Output: undefined
Here, myVar
is created but has no value. So, it’s undefined
.
Now imagine you have an array, and it has undefined
values like this:
let myArray = [1, undefined, 3, undefined, 5]
console.log(myArray) // Output: [1, undefined, 3, undefined, 5]
We don’t like these undefined
values in our array because they don’t help us. Let’s learn how to clean them.
Why Remove Undefined Values?
Here are some reasons:
- Clean Data: A clean array looks better and is easier to use.
- No Errors:
Undefined
values can sometimes cause problems in calculations or when displaying data. - Professional Work: When your code is clean, it looks more professional.
How to Remove Undefined from an Array
There are many ways to remove undefined
values. I will show you 3 simple methods. Choose the one you like the most!
1. Using the .filter()
Method
The .filter()
method is very easy to use. It checks every value in the array and keeps only the values you want.
Example:
let myArray = [1, undefined, 3, undefined, 5]
let cleanArray = myArray.filter((item) => item !== undefined)
console.log(cleanArray) // Output: [1, 3, 5]
How It Works:
.filter()
looks at each item in the array.- It keeps only the items that are not
undefined
. - The result is a new array without
undefined
values.
2. Using a for
Loop
If you are a beginner, you might feel comfortable with a loop. Here is how you can use a for
loop:
Example:
let myArray = [1, undefined, 3, undefined, 5]
let cleanArray = []
for (let i = 0; i < myArray.length; i++) {
if (myArray[i] !== undefined) {
cleanArray.push(myArray[i])
}
}
console.log(cleanArray) // Output: [1, 3, 5]
How It Works:
- The loop goes through each item in
myArray
. - It checks if the item is not
undefined
. - If the item is valid, it adds it to
cleanArray
.
3. Using .reduce()
If you like to experiment, try the .reduce()
method. It collects valid values into one array.
Example:
let myArray = [1, undefined, 3, undefined, 5]
let cleanArray = myArray.reduce((acc, item) => {
if (item !== undefined) {
acc.push(item)
}
return acc
}, [])
console.log(cleanArray) // Output: [1, 3, 5]
How It Works:
.reduce()
starts with an empty array ([]
).- It adds only the valid items (not
undefined
) to the array.
Real-Life Example
Imagine you have a list of scores from a school test. Some scores are missing (undefined
). You want to calculate the average score.
Example:
let scores = [85, undefined, 90, undefined, 88]
let validScores = scores.filter((score) => score !== undefined)
console.log(validScores) // Output: [85, 90, 88]
Now, you can use validScores
to find the average or show them to others.
Tips for Beginners
-
Start with
.filter()
:
It is the easiest method to removeundefined
values. -
Practice Loops:
Learning loops will help you build logic for coding. -
Fix the Problem at the Source:
If you see too manyundefined
values, check why they are there. Sometimes, the problem is with the data source, not the code.
Bonus: Remove null
and NaN
Too
Sometimes, an array may have other bad values like null
or NaN
. Here’s how to clean them all:
Example:
let myArray = [1, undefined, null, NaN, 3]
let cleanArray = myArray.filter((item) => item != null && !isNaN(item))
console.log(cleanArray) // Output: [1, 3]
Explanation:
item != null
removes bothnull
andundefined
.!isNaN(item)
removesNaN
.
Conclusion:
Removing undefined
values from an array is very simple. Use .filter()
, a for
loop, or .reduce()
to make your array clean.
Keep practicing, and you’ll get better every day.
Remember:
JavaScript is not hard—it just takes time and effort to learn. 😊