Published
- 4 min read
JavaScript localStorage: A Beginner’s Guide
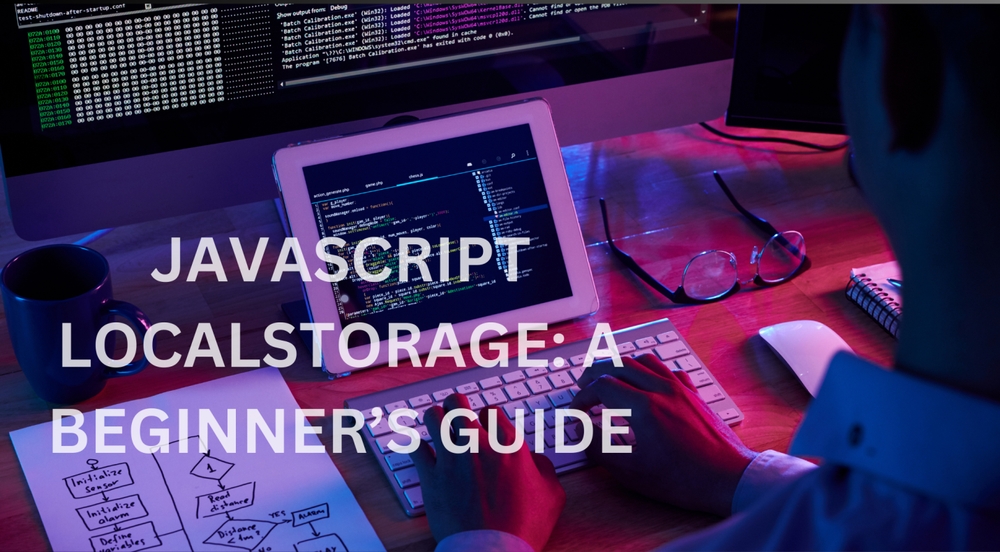
Imagine you’re building a website and want to save some data in the user’s browser, like their name, theme preference (light or dark), or cart items. Instead of saving this data on a server, you can store it directly in the user’s browser using localStorage
in JavaScript. It’s simple, fast, and doesn’t depend on server interaction. Let’s break it down in an easy way.
What is localStorage?
localStorage
is like a small box in your browser where you can keep data as key-value pairs. The best part? The data stays there even after the user closes the browser, unlike temporary storage (like sessionStorage
).
Key Points:
- Persistent Storage: The data remains until you manually remove it.
- Size Limit: You can store up to 5MB in most browsers.
- Key-Value Format: Think of it as a dictionary—each piece of data has a “key” (name) and a “value” (data).
- JavaScript-Only: It works completely on the browser side, no server required.
Why Use localStorage?
- No Server Dependency: No need to make a server request for small things like user preferences.
- Data Stays Persistent: Unlike
sessionStorage
(cleared after the browser is closed),localStorage
data stays until you delete it. - Cross-Page Availability: Data stored in
localStorage
can be accessed across any page of your website. - Super Simple: Just a few lines of code to get started!
How to Use localStorage (With Examples)
Here’s how you can use localStorage
in your projects:
1. Storing Data
To save data, use localStorage.setItem(key, value)
.
// Save user data in localStorage
localStorage.setItem('username', 'naina')
console.log('Data saved!')
Here, username
is the key and naina
is the value.
2. Retrieving Data
To get the stored data, use localStorage.getItem(key)
.
// Retrieve data from localStorage
const username = localStorage.getItem('username')
if (username) {
console.log(`Welcome back, ${username}!`)
} else {
console.log('No user data found.')
}
If the data exists, the app will greet the user.
3. Updating Data
To update the value of a key, just use `setItem()“ again with the same key.
// Update the user's name
localStorage.setItem('username', 'ananya')
console.log('Data updated successfully!')
This will replace the previous value (naina
) with the new value (ananya
).
4. Removing Specific Data
To delete specific data, use localStorage.removeItem(key).
// Remove specific data
localStorage.removeItem('username')
console.log('Data removed!')
5. Clearing All Data
If you want to delete everything stored in localStorage
, use localStorage.clear()
.
// Clear everything
localStorage.clear()
console.log('All data cleared!')
A Practical Example: Saving a Theme Preference
Let’s say you’re building a website with light and dark themes. You can save the user’s theme choice so it loads the same next time.
// Save theme preference
function saveThemePreference(theme) {
localStorage.setItem('theme', theme);
console.log(Theme set to ${theme});
}
// Load theme preference
function loadThemePreference() {
const theme = localStorage.getItem('theme');
if (theme) {
document.body.className = theme; // Apply theme
console.log(Loaded ${theme} theme);
} else {
console.log('No theme preference found');
}
}
// Example usage
saveThemePreference('dark'); // Save 'dark' theme
loadThemePreference(); // Load the theme next time
Best Practices for localStorage
- Don’t Store Sensitive Data: Avoid saving things like passwords or payment info—
localStorage
isn’t secure and can be easily accessed by anyone using developer tools. - Use JSON for Complex Data: If you need to store arrays or objects, convert them to a string using
JSON.stringify()
, and convert back withJSON.parse()
.
// Save an object
const user = { name: 'naina', age: 19 }
localStorage.setItem('user', JSON.stringify(user))
// Retrieve the object
const retrievedUser = JSON.parse(localStorage.getItem('user'))
console.log(retrievedUser.name) // Output: naina
- Check for Browser Support: Not all browsers (especially older ones) fully support
localStorage
. Always check before using it:
if (typeof localStorage !== 'undefined') {
console.log('localStorage is supported!')
} else {
console.log('localStorage is not supported.')
}
- Handle Errors Gracefully: Always handle cases where the data might not be available or properly set.
Common Use Cases
- Saving Preferences: Themes, languages, or layout options.
- Cart Data: Temporarily store items in an e-commerce cart.
- Form Inputs: Preserve user input in case they refresh the page.
Browser Compatibility
localStorage
is supported in modern browsers like Chrome, Firefox, Safari, and Edge. However, some older browsers like Internet Explorer 8 or below might not fully support it.
Conclusion
localStorage
is a powerful tool for storing data on the client side, perfect for small-scale applications. By following the examples and best practices above, you can easily add features like saved preferences, temporary cart storage, or cached data to your projects.