Published
- 2 min read
How to Play a .mp3 File in JavaScript
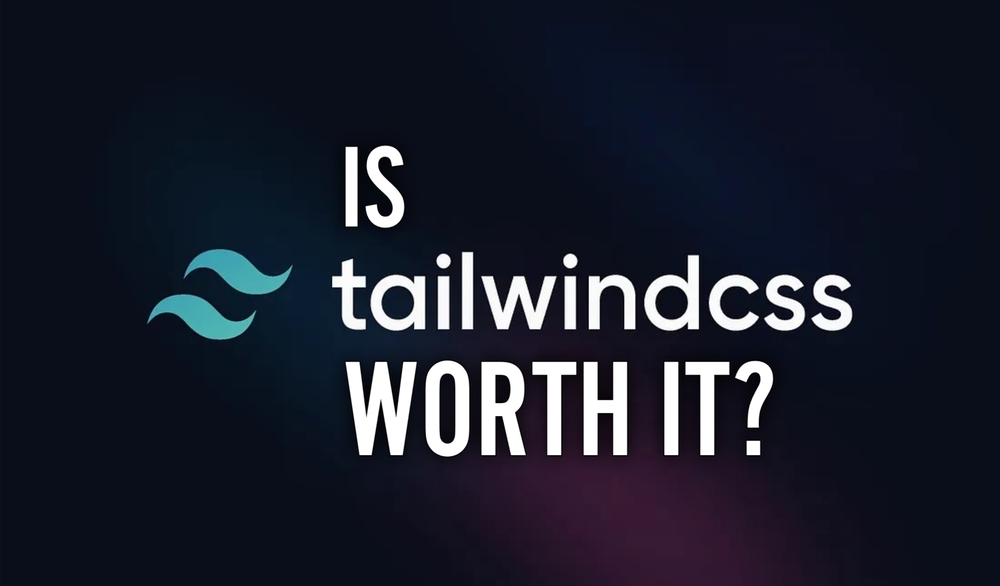
JavaScript provides powerful tools for handling audio, including the ability to play .mp3
files directly in a web application. In this tutorial, we’ll explore how to use JavaScript to play audio files, focusing on the most effective techniques, best practices, and common use cases.
Why Play .mp3 Files in JavaScript?
Playing .mp3
files with JavaScript opens the door to creating dynamic and interactive web applications. Here are some examples:
- Background Music: Add ambiance to games or websites.
- Audio Feedback: Provide sound cues for user interactions.
- Learning Platforms: Play audio lessons or pronunciation guides.
- Media Players: Build custom audio players for users.
Tip: Read through the examples, experiment with the code, and try integrating audio functionality into your projects for hands-on learning.
Methods to Play .mp3 Files
JavaScript allows you to play audio using the <audio>
element or the Audio API. Let’s look at these methods in detail.
1. Using the <audio>
Element
The <audio>
element in HTML provides a straightforward way to embed and control audio.
Basic Example:
<audio id="audioPlayer" src="example.mp3"></audio>
<button onclick="playAudio()">Play</button>
<button onclick="pauseAudio()">Pause</button>
<script>
const audio = document.getElementById('audioPlayer');
function playAudio() {
audio.play(); // Play the audio
}
function pauseAudio() {
audio.pause(); // Pause the audio
}
</script>
Output:
- Clicking Play starts the audio.
- Clicking Pause stops the playback.
2. Using the JavaScript Audio Object
The Audio API offers a more programmatic way to play audio without needing an <audio> element in your HTML.
Basic Example:
const audio = new Audio('example.mp3');
function playAudio() {
audio.play(); // Play the audio
}
function pauseAudio() {
audio.pause(); // Pause the audio
}
Steps:
- Create a new Audio object and pass the .mp3 file’s path as an argument.
- Use the .play() method to start the audio.
- Use the .pause() method to stop the audio.
Advanced Features
Both methods provide ways to customize your audio playback:
Setting Volume
You can set the volume with the .volume property:
audio.volume = 0.5; // Set volume to 50%
Looping Audio
Enable looping with the .loop property:
audio.loop = true; // Audio will repeat indefinitely
Monitoring Playback
Track the playback time using the .currentTime property:
console.log(audio.currentTime); // Log the current playback time
Conclusion
JavaScript makes it simple to add audio to your web applications, whether through the <audio> element or the Audio object. Try out the examples above and experiment with customizations to build engaging, sound-enhanced applications!