Published
- 4 min read
How to Check If a Character is a Double Quote in JavaScript
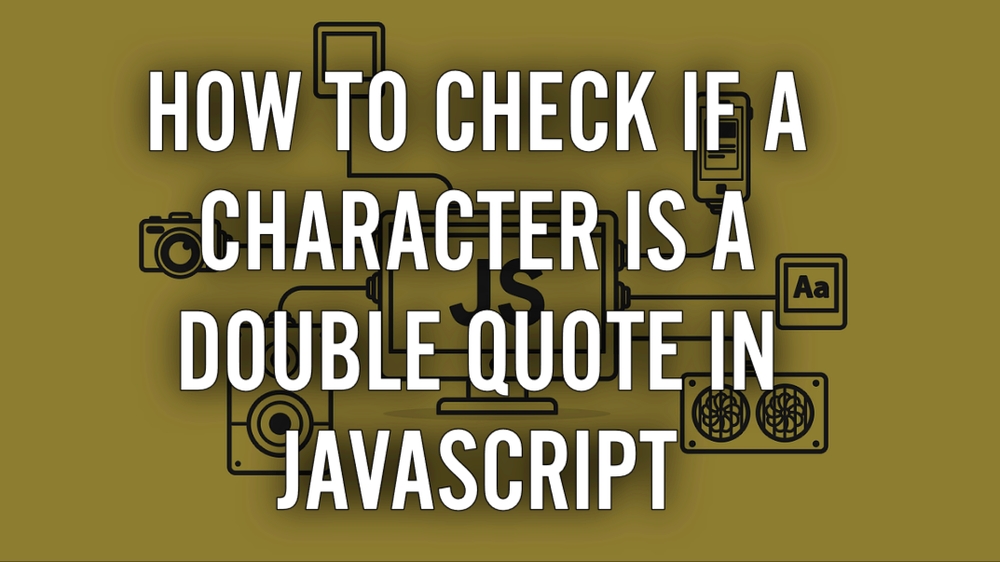
Double quotes ("
) are among the most commonly used characters in JavaScript, primarily to define string literals. However, there may be cases where you need to verify whether a specific character in a string is a double quote. This comprehensive tutorial will guide you step by step through different methods to achieve this, making it easy for beginners to understand.
Why Check for Double Quotes?
Before diving into the implementation, let’s first understand why you might need to check for double quotes in JavaScript:
- Validation: Ensure inputs, such as JSON strings, are properly formatted.
- Sanitization: Remove unwanted characters, like quotes, from user inputs.
- Parsing: Create custom parsers for data formats.
- Debugging: Identify issues in strings caused by misplaced or extra quotes.
Tip: Read this tutorial thoroughly, experiment with the examples, and practice modifying the code to strengthen your understanding.
Basic Concept
In JavaScript, a double quote is simply a character represented by the string literal “. You can compare it with other characters using equality checks. Here’s how it works in the simplest form:
const char = '"'
if (char === '"') {
console.log('The character is a double quote!') // Output: The character is a double quote!
} else {
console.log('The character is not a double quote.')
}
Step-by-Step Explanation
1. Accessing a Character in a String
In JavaScript, strings are iterable, meaning you can access individual characters using their indices:
const str = 'Hello "World"!'
const char = str[6] // Access the character at index 6
console.log(char) // Output: "
2. Comparing the Character
Once you have the character, you can compare it to the double-quote literal:
if (char === '"') {
console.log("It's a double quote.") // Output: It's a double quote.
}
3. Checking All Characters in a String
If you need to check every character in a string for double quotes, you can loop through the string:
Using a for Loop
const str = 'She said, "Hello!"'
for (let i = 0; i < str.length; i++) {
if (str[i] === '"') {
console.log(`Double quote found at index ${i}`) // Output: Double quote found at index 10
}
}
Using the for…of Loop
for (const char of str) {
if (char === '"') {
console.log('Found a double quote!') // Output: Found a double quote!
}
}
4. Counting Double Quotes in a String
If you want to count the number of double quotes in a string, use the following approach:
const str = 'This is a "test" with "double quotes".'
let count = 0
for (const char of str) {
if (char === '"') {
count++
}
}
console.log(`The string contains ${count} double quotes.`) // Output: The string contains 2 double quotes.
5. Using Regular Expressions
Regular expressions provide a powerful way to search for specific patterns in a string, including double quotes:
Finding All Double Quotes
const str = 'This "is" a "test".'
const matches = str.match(/"/g) // Match all double quotes
if (matches) {
console.log(`Found ${matches.length} double quotes.`) // Output: Found 2 double quotes.
} else {
console.log('No double quotes found.')
}
Checking If a String Contains Any Double Quote
const containsDoubleQuote = /"/.test(str)
if (containsDoubleQuote) {
console.log('The string contains a double quote.') // Output: The string contains a double quote.
} else {
console.log('No double quotes in the string.')
}
Edge Cases to Consider
- Empty Strings: Always check for empty strings to avoid unnecessary operations.
const str = ''
if (str === '') {
console.log('The string is empty.') // Output: The string is empty.
}
- Escaped Double Quotes: In some cases, double quotes might be escaped using a backslash (\”). Regular expressions or string methods might handle these differently.
const str = 'He said, \\"Hello\\".'
console.log(str) // Output: He said, \"Hello\".
- Different Encodings: Ensure the text encoding is consistent to avoid unexpected results.
Performance Considerations
When working with large strings, consider the efficiency of your approach:
- Loops: Good for simple checks.
- Regular Expressions: Can simplify complex searches but may have performance overhead.
- String Methods: Functions like
indexOf()
orincludes()
are optimized for specific use cases.
Suggestion: Experiment with these methods on different strings and evaluate their performance, especially when working with large datasets.
Conclusion
Checking if a character is a double quote in JavaScript is straightforward but can vary based on your specific needs. Whether you’re validating inputs, counting occurrences, or parsing text, this guide provides all the tools you need to handle double quotes effectively.
Takeaway: Practice the examples provided, tweak the code to suit your use cases, and don’t hesitate to explore new methods like regular expressions.
By combining techniques such as indexing, loops, and regular expressions, you can efficiently handle even the most complex string operations involving double quotes.