Published
- 4 min read
How to Check if a File Exists in JavaScript – Simple Guide for Beginners
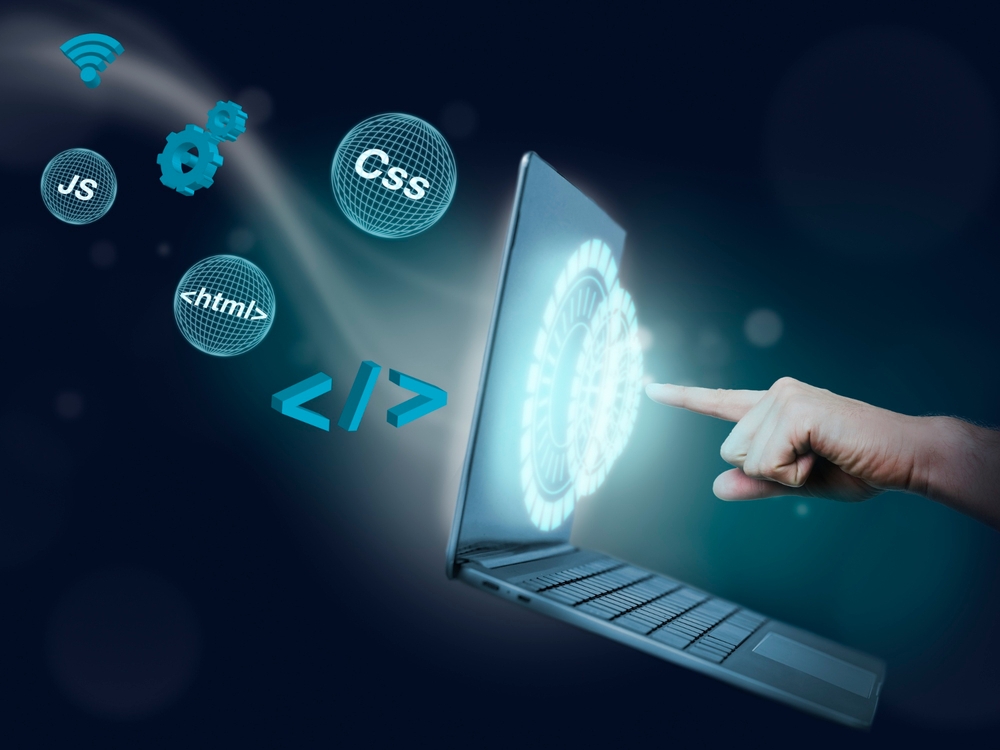
Hey! 😊 Are you just starting with JavaScript? Do you want to know how to check if a file exists? No problem! I’m here to explain it in a simple way, so even if you’re a beginner or your English is still getting better, you will understand easily. Let’s get started!
What Does “Check if a File Exists” Mean?
Before jumping into the code, let’s talk about what it means to check if a file exists. Imagine you’re building a website or an app. You might need to show a picture, load a file, or get some data from a document. But what if the file you’re looking for isn’t there? If you try to open a file that doesn’t exist, your app might crash or show an error. That’s why we need to check if the file exists before we try to use it.
Can JavaScript Check if a File Exists?
Now, let’s answer a common question: Can JavaScript check if a file exists?
The short answer is: Yes, but it depends on where you’re running JavaScript.
-
If you’re working in a web browser (like Google Chrome), JavaScript can only check if a file exists on a web server (online), not on your computer. This is because the browser keeps your computer’s files safe and doesn’t allow JavaScript to see them directly.
-
But if you’re using Node.js (a type of JavaScript that runs on your computer or a server), you can check if a file exists on your computer.
Let’s take a look at both situations with examples.
Method 1: Using Node.js to Check if a File Exists
If you are using Node.js, it’s easier to check if a file exists on your computer or server. Here’s how you can do it.
Example 1: Checking File with fs
(File System) in Node.js
In Node.js, we use a tool called fs (File System) to check for files. Let me show you how:
const fs = require('fs') // This loads the tool to work with files
let filePath = 'path/to/your/file.txt' // Replace this with the file you want to check
fs.access(filePath, fs.constants.F_OK, (err) => {
if (err) {
console.log('The file does not exist')
} else {
console.log('The file exists')
}
})
What’s Happening Here?
- We use
require('fs')
to load the File System tool in Node.js. This tool lets us work with files. - The
fs.access()
function is used to check if the file is there or not. fs.constants.F_OK
tells the program to check if the file exists. It doesn’t open it; it just checks.- If the file doesn’t exist, we get an error, and the message “The file does not exist” is shown.
- If the file does exist, we see the message “The file exists.”
Important
- This method works only in Node.js (for servers). It will not work in a web browser. In the browser, JavaScript can’t access your computer files for security reasons.
Method 2: Checking if a File Exists in a Web Browser
If you’re working in a web browser, JavaScript can’t check files on your computer. But you can still check if a file exists on a website. For example, you can check if an image or a document is available on the web.
Example 2: Checking File with fetch()
in the Browser
In the browser, we use fetch()
to check if a file exists on a web server (like checking if an image exists on a website). Here’s an example:
let fileUrl = 'https://example.com/path/to/your/file.txt' // The file you want to check
fetch(fileUrl)
.then((response) => {
if (response.ok) {
console.log('The file exists on the server')
} else {
console.log('The file does not exist on the server')
}
})
.catch((error) => {
console.log('There was an error with the request')
})
What’s Happening Here?
- The browser checks if the image exists.
- If it’s there, the image is displayed.
- If not, a default image is shown instead.
This is useful because it makes sure your app doesn’t look broken. Even if the file is missing, your users will still see something.