Published
- 3 min read
Beginner's Guide to React Routing with Examples
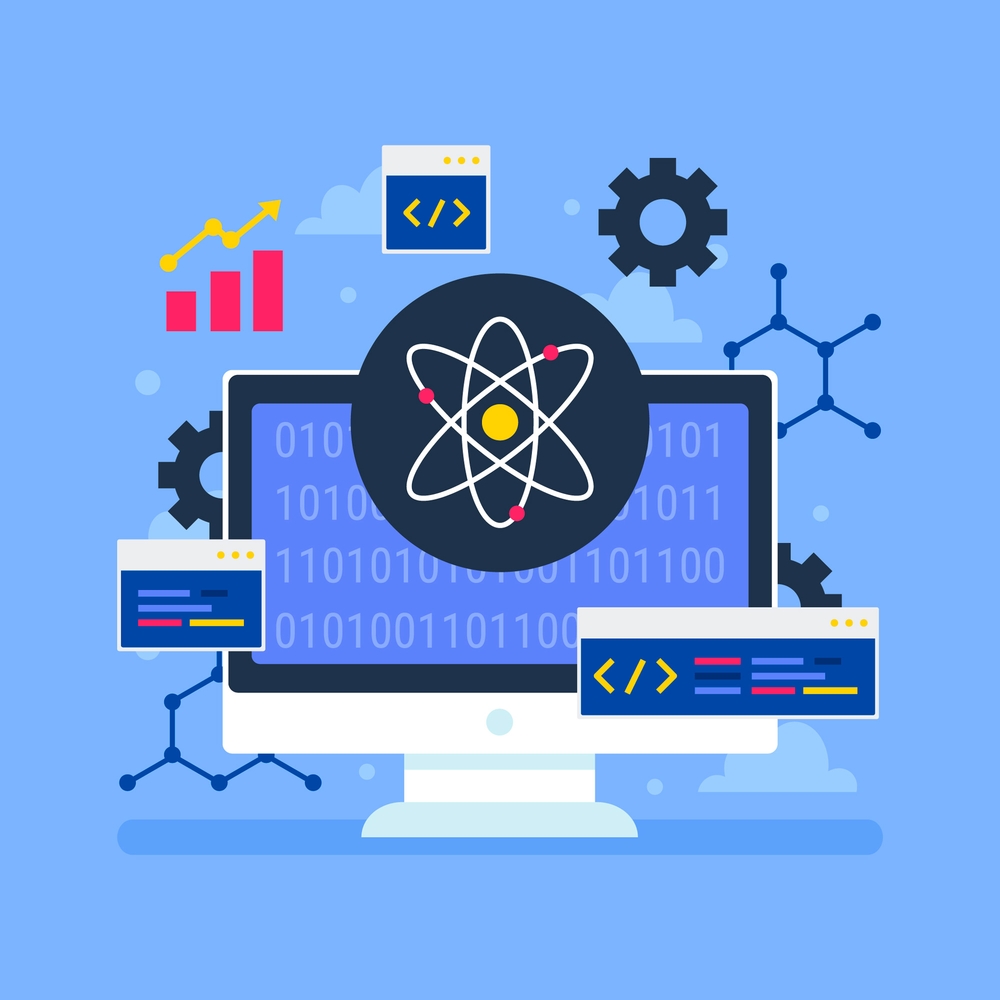
Hi, I’m learning React, and I want to share what I’ve learned about React routing so far. If you’re like me, starting out and feeling a bit confused, don’t worry. I’ll explain everything step by step in easy language.
What is Routing in React?
I will share what I understand about routing. Don’t worry, I’ll explain it in very simple words.
- If you click on “Home,” it shows the Home page.
- If you click on “About,” it takes you to the About page
How to Start with React Router?
Step 1: Install React Router
First, we need to install the library. Don’t worry, it’s easy. Just open your terminal and type:
npm install react-router-dom
Step 2: Import the Required Components
To use React Router, we need to import a few things:
import { BrowserRouter, Routes, Route } from 'react-router-dom'
Here’s what these do:
BrowserRouter:
It wraps the whole app to enable routing.Routes:
It contains all the pages of your app.Route:
It defines each page (e.g., Home, About).
Example: Basic Routing in React
Let’s make a small app with two pages:
Step 1: Create Components Home and About.
First, create two simple components for the pages:
Home.js
function Home() {
return <h1>Welcome to the Home Page</h1>
}
export default Home
About.js
function About() {
return <h1>Welcome to the About Page</h1>
}
export default About
Step 2: Set Up Routing
Now we’ll use React Router in App.js
to set up the routes.
App.js
import React from 'react'
import { BrowserRouter, Routes, Route } from 'react-router-dom'
import Home from './Home'
import About from './About'
function App() {
return (
<BrowserRouter>
<Routes>
<Route path='/' element={<Home />} />
<Route path='/about' element={<About />} />
</Routes>
</BrowserRouter>
)
}
export default App
How It Works
path="/"
: This is the route for the Home page. When you go to `/“, it will show the Home component.path="/about"
: This is the route for the About page. When you go to/about
, it will show the About component.
How to Navigate Between Pages?
We use the Link component to move between pages. It works like an anchor tag, but it doesn’t reload the page.
Step 1: Import Link
In App.js
, import the Link
component:
import { Link } from 'react-router-dom'
Step 2: Add Links to Your App
Now, modify App.js
to include navigation links:
function App() {
return (
<BrowserRouter>
<nav>
<Link to='/'>Home</Link> | <Link to='/about'>About</Link>
</nav>
<Routes>
<Route path='/' element={<Home />} />
<Route path='/about' element={<About />} />
</Routes>
</BrowserRouter>
)
}
Here’s what I did to understand:
- I started with just two pages to keep things simple.
- I typed the code step by step instead of copying it all at once.
- I used
console.log
to see if my components were loading correctly.
Tips for Beginners
- Start small: Just 2–3 pages like Home, About, and Contact.
- Check the URL: After clicking on links, the URL should change (e.g., /about).
- Fix errors: If a route doesn’t work, check for typos in the path or component name.
Conclusion
I also learned how the Link component makes navigation smooth without refreshing the page. It’s like an anchor tag, but better for React apps.
For beginners like me, it’s important to check the URL after clicking the links and look for typos if something doesn’t work. React routing isn’t hard if you take it one step at a time. Now, I feel more confident and excited to create apps with multiple pages.